组件
vue-codemirror
代码编辑器
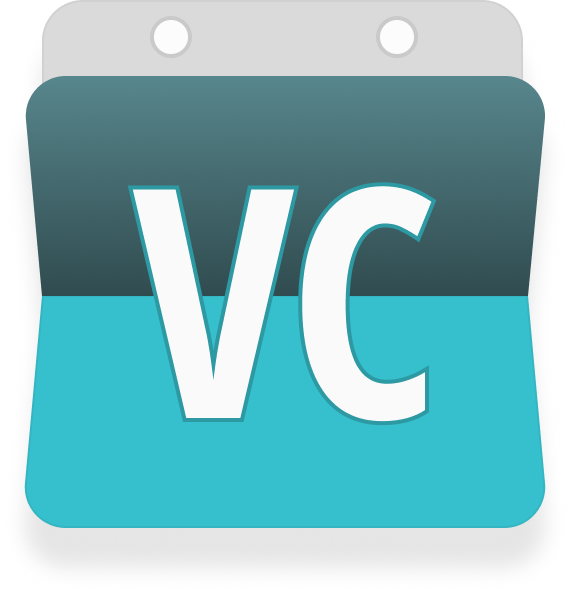
VCalendar
日历
Vue.Draggable
拖拽组件
vue-echarts
二次封装的echarts组件
plotly.js
高层次的、描述性的图表库
vue-katex
Vue2数学公式
- name: vue-codemirror
desc: 代码编辑器
avatar: https://avatars.githubusercontent.com/u/8876537?s=48&v=4
link: https://github.com/surmon-china/vue-codemirror
- name: VCalendar
desc: 日历
avatar: https://res.cloudinary.com/dqgcfqzpk/image/upload/v1557324348/v-calendar/hero.png
link: https://vcalendar.io/
- name: Vue.Draggable
desc: 拖拽组件
avatar: https://sortablejs.github.io/Vue.Draggable/img/logo.c6a3753c.svg
link: https://github.com/ecomfe/vue-echarts/blob/main/README.zh-Hans.md
- name: vue-echarts
desc: 二次封装的echarts组件
avatar: https://avatars.githubusercontent.com/u/2268460?s=48&v=4
link: https://github.com/ecomfe/vue-echarts
- name: plotly.js
desc: 高层次的、描述性的图表库
avatar: https://avatars.githubusercontent.com/u/5997976?s=48&v=4
link: https://plotly.com/javascript/
- name: vue-katex
desc: Vue2数学公式
avatar: https://katex.org/img/katex-logo.svg
link: https://github.com/lucpotage/vue-katex
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
# 应用
vue-echarts
安装
npm install echarts vue-echarts
1npm i -D @vue/composition-api
1引用
import VChart from "vue-echarts";
1做成组件
<!-- VChart --> <script> import VChart from "vue-echarts"; // 必须显式指定此路径而不是默认入口,当前项目webpack打包时默认入口不是module而是main,默认会指向全量引入 import theme from "@/assets/style/echarts/macarons.json"; // 整体主体样式 export default { mixins: [VChart], props: { theme: { type: [String, Object], default: () => theme, }, }, created() {}, }; </script>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16使用
<template>
<div class="echart">
<el-button class="btn" @click="refresh"> 更新数据 </el-button>
<VChart
autoresize
style="width: 100%; height: 75vh"
:option="eChartOptions"
:loading-options="loadingOpt"
:loading="loading"
/>
</div>
</template>
<script>
import VChart from "@/components/echarts/VChart.vue";
const yAxis0 = {
type: "value",
name: "次数",
minInterval: 1,
axisLabel: {
formatter: "{value}",
},
};
const chartSettings = {
eChartOptions: {
tooltip: {
trigger: "axis",
axisPointer: {
type: "line",
},
},
xAxis: {
type: "category",
data: ["Mon", "Tue", "Wed", "Thu", "Fri", "Sat", "Sun"],
},
yAxis: [yAxis0],
series: [
{
data: [150, 230, 224, 218, 135, 147, 260],
type: "line",
},
],
},
updateOptions(data) {
this.eChartOptions.series[0].data = data;
return this.eChartOptions;
},
};
export default {
components: {
VChart,
},
data() {
return {
eChartOptions: null,
loading: false,
loadingOpt: {
text: "loading",
color: "#c23531",
textColor: "#000",
maskColor: "rgba(255, 255, 255, 0.8)",
zlevel: 0,
// Font size. Available since `v4.8.0`.
fontSize: 12,
// Show an animated "spinner" or not. Available since `v4.8.0`.
showSpinner: true,
// Radius of the "spinner". Available since `v4.8.0`.
spinnerRadius: 10,
// Line width of the "spinner". Available since `v4.8.0`.
lineWidth: 5,
// Font thick weight. Available since `v5.0.1`.
fontWeight: "normal",
// Font style. Available since `v5.0.1`.
fontStyle: "normal",
// Font family. Available since `v5.0.1`.
fontFamily: "sans-serif",
},
};
},
mounted() {
this.eChartOptions = chartSettings.eChartOptions;
},
methods: {
refresh() {
this.loading = true;
setTimeout(() => {
this.loading = false;
this.eChartOptions = chartSettings.updateOptions(this.random(7));
}, this.randomInt(100, 500));
},
random(index) {
let arr = []; // 随机数字
for (let i = 0; i < index; i++) {
arr.push(this.randomInt(100, 500));
}
return arr;
},
// 随机数
randomInt(min, max) {
return Math.round(Math.random() * (max - min) + min);
},
},
};
</script>
<style lang="scss" scoped>
.echart {
position: relative;
.btn {
position: absolute;
right: 10%;
z-index: 9;
}
}
</style>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
Plotly.js
安装
yarn add plotly.js-dist
1导入
import Plotly from "plotly.js-dist";
1使用
<template> <div id="graph"></div> </template> <script> import Plotly from "plotly.js-dist"; export default { name: "plotly", data() { return { listData: [], // 数据部分 }; }, mounted() { this.initViolin(this.listData); }, methods: { initViolin(dataList) { // 获取DOM元素 var myPlot = document.getElementById("graph"); let rows = dataList; let data = []; let layout = {}; Plotly.newPlot("graph", data, layout, { showSendToCloud: false }); // 点击图表触发事件 myPlot.on("plotly_click", function (data) { console.log(data); }); }, }, }; </script> <style lang="scss" scoped></style>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
vue-katex
安装
yarn add vue-katex katex
1导入
// 引入vue-katex数学公式 import VueKatex from 'vue-katex'; import 'katex/dist/katex.min.css'; Vue.use(VueKatex, { globalOptions: { //... Define globally applied KaTeX options here } });
1
2
3
4
5
6
7
8
复制代码
编辑 (opens new window)
上次更新: 2025-01-10 13:54:56